Hi I am interested on item 2587. I want to use it, but I don’t find any documentation about the circuit that has to exist for the communication with an arduino uno. I would like to save on it all that I print in the serial monitor (I am using an arduino uno to drive a servo basing on the inclination of an IMU). Can you send me some good links, please?
Hello.
You should not need extra circuitry, since the #2587 has built in voltage dividers. I recommend using the Arduino SD library, which has several helpful examples. The communication between the SD card and the Arduino Uno is done through SPI, and the connections are mentioned in the SD Library documentation and comments of the example codes.
SCLK on the MicroSD breakout board should go to SCK (pin 13) on the Arduino Uno.
DO on the MicroSD breakout board should go to MISO (pin 12) on the Arduino Uno.
DI on the MicroSD breakout board should go to MOSI (pin 11) on the Arduino Uno.
nCS on the MicroSD breakout board depends on your code, but pin 10 is what the SD Library examples use.
Along with those SPI connections, you will need to supply the VDD pin on the board with 5V to power it, and have a common ground connection.
The CD pin can optionally be used as an input to the Arduino to detect whether there is a card inserted. When a card is inserted, it is high; when no card is inserted, it is shorted to ground.
Brandon
Hi @BrandonM I used your setup, paying attention to the connection and the code but it doesn’t work. Where could be the problem? the output is a failed initialization. I tried more microsd card ( in some cases I also formatted them) but the result is always the same
I am using an artduino uno wifi rev 2 and the wiring is:
- gnd-gnd
- vcc-5V
- di-11
- do-12
- sclk-13
- cs-10
and the code is
#include <SPI.h>
#include <SD.h>
File myFile;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
Serial.print("Initializing SD card...");
if (!SD.begin(10)) {
Serial.println("initialization failed!");
while (1);
}
Serial.println("initialization done.");
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
myFile = SD.open("test.txt", FILE_WRITE);
// if the file opened okay, write to it:
if (myFile) {
Serial.print("Writing to test.txt...");
myFile.println("This is a test file :)");
myFile.println("testing 1, 2, 3.");
for (int i = 0; i < 20; i++) {
myFile.println(i);
}
// close the file:
myFile.close();
Serial.println("done.");
} else {
// if the file didn't open, print an error:
Serial.println("error opening test.txt");
}
}
void loop() {
// nothing happens after setup
}
but it doesn’t work.
Btw the goal for me is to use it with a portenta h7 where the SPI connection is with pin 7,8,9,10. Can you confirm it, please?
PS: I supposed that with my arduino it can work well.
Thank you for the additional information. It looks like you are using an Arduino WiFi Rev 2 board, which is different than a normal Arduino Uno. Could you try connecting to the ISP pins on the 2x3 ICSP header instead of pins 11-13? You can refer to this pinout diagram of that header for reference:
So, SCLK should connect to ICSP pin 3, DO to ICSP pin 1, and DI to COPI. You can leave the other connections how they were.
By the way, the library only supports FAT16 or FAT32 file systems, so if you haven’t formatted your card to use one of those, that might also be a problem. I recommend reading through the notes on the library here, which also goes through how to format the card properly. Also, as far as code, I recommend sticking to the examples for now; particularly, the Card Info example, as that one is a good way to confirm that the communication is working.
I am not very familiar with the Portenta H7, but unlike the Arduino Uno, it looks like it operates at 3.3V. For 3.3V applications, we carry a smaller Breakout Board for MicroSD Card without the 3.3 V regulator, level shifters, and mounting holes. As far as the connections, it looks like Arduino’s documentation lists pins 7, 8, 9, and 10 as SPI pins CS, COPI, CK, and CIPO, respectively.
Brandon
Hi @BrandonM I hope you spent nice holidays.
Thank you so much with the connection you said me the board find the card. By the way, after reading notes, I formatted my sd. The problem now is that it didn’t find (in CardInfo.ino) the right partition even if it is a default characteristics of my sd. Do you have some tip for me?
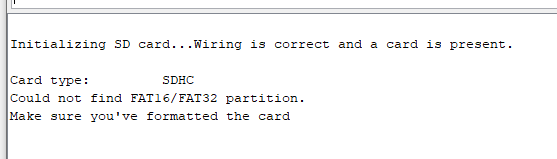
You might double check that you are using the latest version of the SD library (you can update it from the library manager within the Arduino IDE).
I have also seen people claiming that switching to this SdFat library, which has a similar SdInfo example, solved the problem.
If you have any other micro SD cards you could try (preferably lower capacity like 2GB), that would be a good test too.
Brandon
Hi Brandon and thank you so much. I finally figure out with SD library. Right now I have another problem unfortunately. I hope that you kindly can explain me and help me to solve it. If I create a file named “salva.txt”, the output in the serial monitor is repeated only once (right), but if I later go to the saved file the output over there is written twice. I found other problems like this but no solution.
#include <SPI.h>
#include <SD.h>
File myFile;
int CS_PIN = 10;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
Serial.print("Initializing SD card...");
if (!SD.begin(10)) {
Serial.println("initialization failed!");
while (1);
}
Serial.println("initialization done.");
// open the file. note that only one file can be open at a time,
// so you have to close this one before opening another.
myFile = SD.open("salva.txt", FILE_WRITE);
// if the file opened okay, write to it:
if (myFile) {
Serial.print("Writing to test.txt...");
myFile.println("This is a test file :)");
myFile.println("testing 1, 2, 3.");
for (int i = 0; i < 20; i++) {
myFile.println(i);
Serial.println(i);
}
// close the file:
myFile.close();
Serial.println("done.");
} else {
// if the file didn't open, print an error:
Serial.println("error opening test.txt");
}
}
void loop() {
// nothing happens after setup
}
salva.txt:
This is a test file
testing 1, 2, 3.
0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
This is a test file
testing 1, 2, 3.
0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
The Arduino Uno boards reset when you open the Serial Monitor, so what I suspect is happening is that when you connect/power your Arduino, it writes to the SD card, then when you open the Serial Monitor, it writes everything again. You can test this by adding (correction in post below)while(!Serial);
after your Serial.begin(9600);
command to make the code only progress once you open the Serial Monitor.
Also, please note that the file will just be added onto if you are not manually erasing it after each time you run the program.
Brandon
Hello, again.
Actually, another engineer here pointed out that while(!Serial);
will probably not work with the Arduino Uno the way it works with the Leonardo (and our A-Star boards), but I still suspect resetting when the Serial Monitor starts up is what you are running into. Probably the easiest way to test this would be to add a line of code to turn on the user LED and indicate when the program is done writing to the microSD card. Then you can just power it up, wait for the LED to come on, and power it down to eject the microSD card and check what was written to it (without ever opening the Serial Monitor).
Brandon
Thank you for your disponibility and your kindness. I confirm your problem’s theory. I am thinking that, in order to have a debug in the serial monitor, I shall open it and drive with a remote control the initialization and writing of the sd. In this way, at the first power on the sd card shall still waiting for a command.
Matteo
Hey @BrandonM thank you again for your previous support. It lets me to conduct more test and it worked good. I am writing again to you searching for piece of code I would say: by means of a Bluetooth communication I would like to send, to vary, the name of the file that the SD has to create. I am able to send Strings but they are not acceptable from the sd library. the error is : cannot convert ‘arduino::String’ to ‘char*’ for argument ‘1’ to ‘void createfile(char*)’ and I saw it more times testing more solutions. Indeed I am not so skilled with char array.
How can I do that using char array o c-strings? Can you help me please?
We do not have any example code for naming files on an SD card from a Bluetooth connection, but my understanding is that the SD library should accept strings as filenames. I do not see any createfile()
function in the SD library; is that a function you wrote yourself? Could you post a simplified, but complete program that demonstrates the problem?
Brandon
Sure Brandon I was not too complete. This is the section of code with the focus on my problem. I commented the conversion of my long string into a char. I tested it in order to use the function created to name a file where the data have to be saved. I tried to give also a String as input but I found on web that it is not admitted. Can you give me some clearness, please? My desire is to create each time that I send the command “name” a different filename (s,t,c,r are always the same) Thank You.
#include <SoftwareSerial.h>
SoftwareSerial BT(12, 13);
/**** SD ****/
#include <Wire.h>
#include <SPI.h>
#include <SD.h>
const uint8_t CS_PIN = 4;
File file;
String line, title; bool state_name = 0; bool command = 0;
#define s "task1"
#define t "task2"
#define c "task3"
#define r "task4"
#define format ".txt"
//char title[];
void setup() {
/**** COMMUNICATION ****/
Serial.begin(57600);
BT.begin(57600);
BT.println() ;
BT.println() ;
Wire.begin();
/**** SD ****/
initializeSD();
delay(1000);
Serial.println("SETUP DONE");
}
void loop() {
//read command from Bluetooth
if (BT.available()) line = BT.readStringUntil('\n');
/****ID code****/
if (line.startsWith("name") && state_name == 0 ) {
state_name = 1;
title = s + line.substring(4, 6) + t + line.substring(6, 8) + c + line.substring(8, 10) + r + line.substring(10, 12) + ".txt";
// title length: 30 +0 position
// char charBuf[31];
// title=title.toCharArray(charBuf, 31)
//
// title= title.c_str();
//const char * title=title.c_str();
Serial.print("ID = "); Serial.println(title);
// BT.print("ID = "); BT.println(title);
}
if (command == 0 && line.startsWith("LON")) {
// createfile("test.txt");
createfile(title);
file.println(millis());
file.close();
}
if (command == 0 && line.startsWith("LOFF")) {
Serial.println("FINISH"); BT.println("FINISH!");
createfile(title);
file.println(F("FINISH"));
file.close();
command = 0;
state_name = 0;
}
}
/* Init SD */
void initializeSD()
{
//Serial.println();
//Serial.println("Initializing SD card...");
pinMode(CS_PIN, OUTPUT);
if (SD.begin())
{
Serial.println("SD card is ready to use.");
BT.println("SD card is ready to use.");
} else
{
Serial.println("SD card initialization failed");
BT.println("SD card initialization failed");
return;
}
}
void createfile(char filename[] )
//void createfile(String filename )
{
file = SD.open(filename, FILE_WRITE);
if (file)
{
//Serial.println("File created successfully.");
return 1;
} else
{
Serial.println("Error while creating file.");
BT.println("Error SD");
return 0;
}
}
Thank you for the additional information.
I do not see a problem with using a string as an argument to your function; I am not sure what you mean when you say it is “not admitted”. However, you might consider changing it so the string is passed by reference instead (i.e. void createFile(String &filename)
) to avoid making an unnecessary copy of it.
Brandon
Thank you for the reply, first of all. I was thinking the same,but continuing to testing, I noticed today that the real problem is the length. I can’t create a filename with length>8. It is annoying but I can survive let’s say.