TX stands for Transmit, and RX stands for Receive, so in general when you want two devices to communicate, you want to connect TX from one device to RX from another. This is an odd case, since usually you want to connect pins with the same label together (like GND to GND).
You could think of your mouth as being a TX pin, and your ears as being an RX pin. If we’re standing in different rooms, we can talk to each other with two sets of those tin can string-phones, which are just like wires. Of course, the string phone from my mouth should go to your ear, and the one from your mouth should go to my ear. It’s not very useful to string our ears together.
Extending the comparison a little, multiple devices can receive instructions from a single commanding device by splitting a wire from the commanding device’s TX pin to the RX pins of the receiving devices, or:
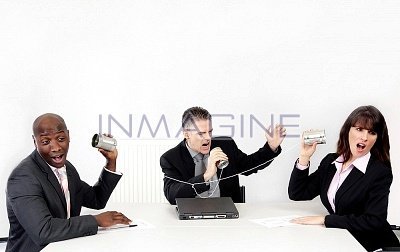
TX and RX pin labeling can be really confusing though. Usually pins are labeled from the point of view of the device itself, so a TX label means “This is my TX pin” (all Pololu devices are labeled this way). Sometimes there is a distinction made between a master device and a slave device, and while TX on the master device means “This is my TX pin”, TX on the slave device may mean “This is my TX pin” or it may mean the opposite “Connect the master’s TX pin here, this is my RX pin”.
To further complicate things, serial cables can be wired differently. Some 9-pin serial cables are “straight through,” meaning pin 2 at one end connects to pin 2 at the other end, and the same goes for pin 3. These are meant for connecting a master device, or DTE (Data Terminal Equipment, i.e. your computer) to a slave device, or DCE (Data Circuit-terminating Equipment, i.e. an old serial modem). The computer sends on pin 3 and receives on pin 2, and the modem is wired already to send on pin 2 and receive on pin 3. A straight-through cable connects pin 2 to pin 2, and pin 3 to pin 3, and both devices are happy. There are also “null-modem” cables, which are meant to connect two master devices, like two computers, together. Since both devices are expecting to transmit on pin 2 and receive on pin 3, the wires are crossed inside the cable (pin 2 at one end goes to pin 3 at the other). It gets really crazy. The first thing I do when I pick up a serial cable is test the wiring.
It generally does not work to have multiple TX pins wired together without some extra components, and you would need to make sure that the connected transmitters try to talk at the same time. If all you want to do is command the motors, you can leave the TX pins on your two TReX controllers disconnected (you will need to have a controller’s TX pin connected when you set it up with the configurator program). If you need to use the response-features of your TReX controllers (like if you wanted to measure the motor current for example) I would suggest just getting two USB-to-Serial adapters.
ANYWAY, that servo control code I sent you builds strings of bytes and then sends them out a serial port, but you can totally try strings out with Br@y terminal first. You can type byte values into the white text box near the bottom to build up strings of bytes, then click “send” when you’re ready to transmit them (you should uncheck the “+CR” box, which optionally adds a carriage return, byte value 13, to the end of each command).
To build commands in hex, start with a $ character followed by two hex digits. So, if you wanted to send the string [128,1,4,1,23,63] you would type “$80$01$04$17$3F”. You can send decimal numbers by starting with a # character followed by three dec digits, so to send the same string [128,1,4,1,23,63] you would type “#128#001#004#001#023#063”. You can mix and match too, like this: “$80$01$04#001#023$3F”. Just be sure to always fill up the two hex digits or three dec digits (add leading zeros if you need to) or the terminal program will interpret them as ascii text characters instead.
So, what are your plans for two computer joystick-controlled TrEX motors?
-Adam
P.S. The windows calculator will convert numbers between decimal and hex. Under the “view” menu select “scientific”. You should now have a set of buttons just below the number readout (on the left) that let you switch the displayed number between Hex, Dec, Oct, and Bin. Quite useful.