Hi Kevin, yes that works - many thanks. Such a small thing and a goofy oversight. It wasn’t apparent to me because I run the script as a service and not all of the console messages appear in the service status.
For the purpose of this topic question, I think this can be closed. There is another issue below which has emerged, but I’m happy raising another thread if that’s preferable. This issue might be arising because I am now connecting to the RPI as a closed network wireless client, with hostapd
and dhcpd
configured to broadcast an SSID without Internet connectivity.
I observed your suggested fix (and a very basic one) working once but then something went awry and I can’t seem to right it. Now when I run the server_balboa.py
using the vanilla github code, I get the following error, even after rebooting and resetting the 32U4!
root@rpi3-32U4-pololu:/home/pi/pololu-rpi-slave-arduino-library-balboa/pi# python3 server_balboa.py
Traceback (most recent call last):
File "/usr/lib/python3/dist-packages/flask/app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "/usr/lib/python3/dist-packages/flask/app.py", line 1985, in wsgi_app
response = self.handle_exception(e)
File "/usr/lib/python3/dist-packages/flask/app.py", line 1540, in handle_exception
reraise(exc_type, exc_value, tb)
File "/usr/lib/python3/dist-packages/flask/_compat.py", line 33, in reraise
raise value
File "/usr/lib/python3/dist-packages/flask/app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "/usr/lib/python3/dist-packages/flask/app.py", line 1614, in full_dispatch_request
rv = self.handle_user_exception(e)
File "/usr/lib/python3/dist-packages/flask/app.py", line 1517, in handle_user_exception
reraise(exc_type, exc_value, tb)
File "/usr/lib/python3/dist-packages/flask/_compat.py", line 33, in reraise
raise value
File "/usr/lib/python3/dist-packages/flask/app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "/usr/lib/python3/dist-packages/flask/app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "/home/pi/pololu-rpi-slave-arduino-library-balboa/pi/server_balboa.py", line 124, in hearbeat
a_star.leds(not led0_state, not led1_state, not led2_state)
File "/home/pi/pololu-rpi-slave-arduino-library-balboa/pi/a_star.py", line 42, in leds
self.write_pack(0, 'BBB', red, yellow, green)
File "/home/pi/pololu-rpi-slave-arduino-library-balboa/pi/a_star.py", line 37, in write_pack
self.bus.write_i2c_block_data(SLAVE_ADDRESS, address, data_array)
File "/usr/local/lib/python3.5/dist-packages/smbus/util.py", line 59, in validator
return fn(*args, **kwdefaults)
File "/usr/local/lib/python3.5/dist-packages/smbus/smbus.py", line 275, in write_i2c_block_data
raise IOError(ffi.errno)
OSError: 121
The screen looks like this and the calibrate button doesn’t work. I am mystified.
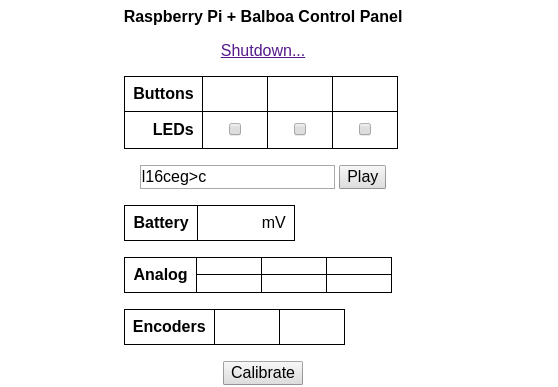